CloudCompare Data Structures & Interfaces
Base
ccObject

- class pycc.ccObject
Bases:
pybind11_object
The base class of all CloudCompare’s data types
ccOjbects have a name that can be changed
an ‘enabled’ state to control if the object should be displayed
a metadata system
Example
>>> import pycc >>> pc = pycc.ccPointCloud() >>> pc.setName("Green Yoshi") >>> pc.getName() == "Green Yoshi" True >>> pc.hasMetaData("Favorite Food") False >>> pc.getMetaData("Favorite Food") is None True >>> pc.setMetaData("Favorite Food", "Apples") >>> pc.hasMetaData("Favorite Food") True >>> pc.getMetaData("Favorite Food") == "Apples" True
- getMetaData(self: pycc.ccObject, key: QString) QVariant
Returns the metadata associated to a key.
Returns None if no metadata for the key is found
- Parameters:
key (str) – The key / name of the metadata to retrieve
- getName(self: pycc.ccObject) QString
Returns the name of the object
- getUniqueID(self: pycc.ccObject) int
- hasMetaData(self: pycc.ccObject, key: QString) bool
Returns True if the object has some metadata associated to the key
- Parameters:
key (str) – The key / name of the metadata to remove
- isCustom(self: pycc.ccObject) bool
- isEnabled(self: pycc.ccObject) bool
Returns whether the object is enabled
- isHierarchy(self: pycc.ccObject) bool
- isLeaf(self: pycc.ccObject) bool
- isLocked(self: pycc.ccObject) bool
Returns whether the object is locked
- removeMetaData(self: pycc.ccObject, key: QString) bool
Removes the metadata associated to a key.
- Parameters:
key (str) – The key / name of the metadata to remove
- setEnabled(self: pycc.ccObject, state: bool) None
Sets the “enabled” state
- Parameters:
state (bool) – The new “enabled” state
- setLocked(self: pycc.ccObject, arg0: bool) None
- setMetaData(self: pycc.ccObject, key: QString, data: QVariant) None
Sets the metadata associated to a key.
- Parameters:
key (str) – The key / name of the metadata to remove
data (str | int) – The value
- setName(self: pycc.ccObject, name: QString) None
Sets the name of the object
- Parameters:
name (str) – The new name
- toggleActivation(self: pycc.ccObject) None
ccHObject

- class pycc.ccHObject
Bases:
ccObject
,ccDrawableObject
CloudCompare’s hierarchical object
Hierarchical objects can have children forming a hierarchy tree
Example
>>> import pycc >>> h = pycc.ccHObject("My Project Hierarchy")
This new hierarchy has no parents, nor children:
>>> h.getParent() is None True >>> h.getChildrenNumber(), h.getChildCountRecursive() (0, 0)
- addChild(self: pycc.ccHObject, child: object, dependencyFlags: pycc.DEPENDENCY_FLAGS = <DEPENDENCY_FLAGS.DP_PARENT_OF_OTHER: 24>, insertIndex: int = -1) bool
- applyGLTransformation_recursive(self: pycc.ccHObject, trans: pycc.ccGLMatrix = None) None
- detachAllChildren(self: pycc.ccHObject) None
- detachChild(*args, **kwargs)
Overloaded function.
detachChild(self: object, child: object) -> None
detachChild(self: pycc.ccHObject, arg0: object) -> None
- find(self: pycc.ccHObject, uniqueID: int) pycc.ccHObject
- getChild(self: object, child: int) object
- getChildCountRecursive(self: pycc.ccHObject) int
Returns the recursive number of children
- getChildIndex(self: pycc.ccHObject, child: pycc.ccHObject) int
- getChildrenNumber(self: pycc.ccHObject) int
Returns the number of ‘direct’ children this object has
- getDisplayBB_recursive(self: pycc.ccHObject, relative: bool, display: ccGenericGLDisplay = None) ccBBox
- getFirstChild(self: pycc.ccHObject) object
- getGLTransformationHistory(self: pycc.ccHObject) pycc.ccGLMatrix
- getIndex(self: pycc.ccHObject) int
- getLastChild(self: pycc.ccHObject) object
- getParent(self: pycc.ccHObject) pycc.ccHObject
Returns the parent of this object
Returns None if the object has no parent
- isAncestorOf(self: pycc.ccHObject, anObject: pycc.ccHObject) bool
- isGroup(self: pycc.ccHObject) bool
- notifyGeometryUpdate(self: pycc.ccHObject) None
Notifies all dependent entities that the geometry of this entity has changed
- resetGLTransformationHistory(self: pycc.ccHObject) None
- setGLTransformationHistory(self: pycc.ccHObject, mat: pycc.ccGLMatrix) None
- swapChildren(self: pycc.ccHObject, firstChildIndex: int, secondChildIndex: int) None
PointCloud
ccGenericPointCloud

- class pycc.ccGenericPointCloud
Bases:
ccShiftedObject
,GenericIndexedCloudPersist
- clear(self: pycc.ccGenericPointCloud) None
- clone(self: pycc.ccGenericPointCloud, desttClous: pycc.ccGenericPointCloud = None, ignoreChildren: bool = False) pycc.ccGenericPointCloud
ccPointCloud
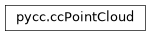
- class pycc.ccPointCloud
Bases:
__ccGenericPointCloudTpl
The main class to represent point clouds in CloudCompare.
Two constructor are available:
- Parameters:
name (str,) – The name of the point cloud
uniqueID (int) – Unique ID, you practically never need to give a value as it is one is already selected by default.
Example
import pycc pc = pycc.ccPointCloud("Yoshi's Island")
- Parameters:
x (numpy.array)
y (numpy.array)
z (numpy.array)
Example
import numpy as np import pycc x = np.array([1, 2, 3]) y = np.array([1, 2, 3]) z = np.array([1, 2, 3]) pc = pycc.ccPointCloud(x, y, z)
- addPoints(self: pycc.ccPointCloud, arg0: numpy.ndarray[numpy.float32], arg1: numpy.ndarray[numpy.float32], arg2: numpy.ndarray[numpy.float32]) None
- colorize(self: pycc.ccPointCloud, arg0: float, arg1: float, arg2: float, arg3: float) bool
- colors(self: pycc.ccPointCloud) object
“Returns the colors as a “view” in a numpy array if the point cloud does not have colors, None is returned
As this is a “view”, modifications made to this will reflect on the point cloud
- computeNormalsWithGrids(self: pycc.ccPointCloud, minTriangleAngle_deg: float = 1.0, pDlg: ccProgressDialog = None) bool
- computeNormalsWithOctree(self: pycc.ccPointCloud, model: cccorelib.LOCAL_MODEL_TYPES, preferredOrientation: ccNormalVectors::Orientation, defaultRadius: float, pDlg: ccProgressDialog = None) bool
- crop2D(self: pycc.ccPointCloud, poly: ccPolyline, orthodDim: int, inside: bool = True) cccorelib.ReferenceCloud
- getCurrentDisplayedScalarField(self: pycc.ccPointCloud) pycc.ccScalarField
- getCurrentDisplayedScalarFieldIndex(self: pycc.ccPointCloud) int
- getPointColor(self: pycc.ccPointCloud, index: int) pycc.Rgba
Returns the color of the point at the given index
Colors must have been enabled before
- getPointNormal(self: pycc.ccPointCloud, index: int) cccorelib.CCVector3
Returns the normal of the point at the given index
- normals(self: pycc.ccPointCloud) object
Returns a copy of the normals into a numpy array
As this is a copy, changes made to this array won’t reflect in the point cloud
- orientNormalsTowardViewPoint(self: pycc.ccPointCloud, VP: cccorelib.CCVector3, pDlg: ccProgressDialog = None) bool
- orientNormalsWithFM(self: pycc.ccPointCloud, level: int, pDlg: ccProgressDialog = None) bool
- orientNormalsWithMST(self: pycc.ccPointCloud, kNN: int, pDlg: ccProgressDialog = None) bool
- partialClone(self: pycc.ccPointCloud, reference: cccorelib.ReferenceCloud) pycc.ccPointCloud
- points(self: pycc.ccPointCloud) numpy.ndarray
- reserveTheNormsTable(self: pycc.ccPointCloud) bool
- reserveThePointsTable(self: pycc.ccPointCloud, _numberOfPoints: int) bool
- reserveTheRGBTable(self: pycc.ccPointCloud) bool
- resizeTheNormsTable(self: pycc.ccPointCloud) bool
- resizeTheRGBTable(self: pycc.ccPointCloud, fillWithWhite: bool = False) bool
- setColor(self: pycc.ccPointCloud, r: int, g: int, b: int, a: int = 255) None
Sets the color for the whole point cloud
- setColors(*args, **kwargs)
Overloaded function.
setColors(self: pycc.ccPointCloud, r: numpy.ndarray[numpy.uint8], g: numpy.ndarray[numpy.uint8], b: numpy.ndarray[numpy.uint8], a: numpy.ndarray[numpy.uint8] = None) -> None
Sets the colors in the point cloud with the given r, g, b arrays (and the optinal alpha array).
Enables colors if not done already
setColors(self: pycc.ccPointCloud, colors: numpy.ndarray[numpy.uint8]) -> None
Sets the colors of the points using the given array.
The array must be of shape (cloud.size(), 3)
Enables colors if not done already
- setCurrentDisplayedScalarField(self: pycc.ccPointCloud, index: int) None
- setNormals(self: pycc.ccPointCloud, arg0: numpy.ndarray[numpy.float32]) None
Sets the normals of the points using the given array.
The array must be of shape (cloud.size(), 3)
Enables normas if not done already
- setPointColor(*args, **kwargs)
Overloaded function.
setPointColor(self: pycc.ccPointCloud, index: int, color: pycc.Rgb) -> None
Sets the color of the point at the given index with the new value
Colors must have been enabled before
setPointColor(self: pycc.ccPointCloud, index: int, color: pycc.Rgba) -> None
Sets the color of the point at the given index with the new value
Colors must have been enabled before
- setPointNormal(self: pycc.ccPointCloud, index: int, normal: cccorelib.CCVector3) None
Sets the normal of the point at the given index with the new value
- setPointSize(self: pycc.ccPointCloud, size: int) None
- sfColorScaleShown(self: pycc.ccPointCloud) bool
- showNormalsAsLines(self: pycc.ccPointCloud, state: bool) None
- showSFColorsScale(self: pycc.ccPointCloud, state: bool) None
- shrinkToFit(self: pycc.ccPointCloud) None
ccPolyline

- class pycc.ccPolyline
Bases:
Polyline
,ccShiftedObject
- computeLength(self: pycc.ccPolyline) float
- getColor(self: pycc.ccPolyline) pycc.Rgb
- getVertexMarkerWidth(self: pycc.ccPolyline) int
- getWidth(self: pycc.ccPolyline) float
- importParametersFrom(self: pycc.ccPolyline, poly: pycc.ccPolyline) None
- is2DMode(self: pycc.ccPolyline) bool
- samplePoints(self: pycc.ccPolyline, densityBased: bool, samplingParameter: float, withRGB: bool) pycc.ccPointCloud
- segmentCount(self: pycc.ccPolyline) int
- set2DMode(self: pycc.ccPolyline, state: bool) None
- setColor(self: pycc.ccPolyline, col: pycc.Rgb) None
- setForeground(self: pycc.ccPolyline, state: bool) None
- setVertexMarkerWidth(self: pycc.ccPolyline, width: int) None
- setWidth(self: pycc.ccPolyline, width: float) None
- showArrow(self: pycc.ccPolyline, state: bool, vertIndex: int, length: float) None
- showVertices(self: pycc.ccPolyline, state: bool) None
- smoothChaikin(self: pycc.ccPolyline, ratio: float, iterationCount: int) pycc.ccPolyline
- split(self: pycc.ccPolyline, maxEdgeLength: float) list[pycc.ccPolyline]
- verticesShown(self: pycc.ccPolyline) bool
Mesh

ccGenericMesh
- class pycc.ccGenericMesh
Bases:
GenericIndexedMesh
,ccHObject
- capacity(self: pycc.ccGenericMesh) int
- getAssociatedCloud(self: pycc.ccGenericMesh) ccGenericPointCloud
- getMaterialSet(self: pycc.ccGenericMesh) ccMaterialSet
- getTexCoordinatesTable(self: pycc.ccGenericMesh) TextureCoordsContainer
- getTriangleMtlIndex(self: pycc.ccGenericMesh, triangleIndex: int) int
- getTriangleNormalIndexes(self: pycc.ccGenericMesh, triangleIndex: int) tuple
- getTriangleNormals(self: pycc.ccGenericMesh, triangleIndex: int) tuple
- getTriangleTexCoordinates(self: pycc.ccGenericMesh, triIndex: int) tuple
- getTriangleTexCoordinatesIndexes(self: pycc.ccGenericMesh, triangleIndex: int) tuple
- hasMaterials(self: pycc.ccGenericMesh) bool
- hasPerTriangleTexCoordinates(self: pycc.ccGenericMesh) bool
- hasTextures(self: pycc.ccGenericMesh) bool
- hasTriNormals(self: pycc.ccGenericMesh) bool
- refreshBB(self: pycc.ccGenericMesh) None
ccMesh
- class pycc.ccMesh
Bases:
ccGenericMesh
- addTriangle(self: pycc.ccMesh, i1: int, i2: int, i3: int) None
Adds a triangle to the mesh Bounding-box validity is broken after a call to this method. However, for the sake of performance, no call to notifyGeometryUpdate is made automatically. Make sure to do so when all modifications are done!
- Parameters:
cloud) (i3 third vertex index (relatively to the vertex)
cloud)
cloud)
- setAssociatedCloud(self: pycc.ccMesh, cloud: ccGenericPointCloud) None
ccSubMesh
- class pycc.ccSubMesh
Bases:
ccGenericMesh
- addTriangleIndex(*args, **kwargs)
Overloaded function.
addTriangleIndex(self: pycc.ccSubMesh, globalIndex: int) -> bool
addTriangleIndex(self: pycc.ccSubMesh, firstIndex: int, lastIndex: int) -> bool
- clear(self: pycc.ccSubMesh, arg0: bool) None
- forwardIterator(self: pycc.ccSubMesh) None
- getAssociatedMesh(self: pycc.ccSubMesh) pycc.ccMesh
- getCurrentTriGlobalIndex(self: pycc.ccSubMesh) int
- getTriGlobalIndex(self: pycc.ccSubMesh, index: int) int
- reserve(self: pycc.ccSubMesh, n: int) bool
- resize(self: pycc.ccSubMesh, n: int) bool
- setAssociatedMesh(self: pycc.ccSubMesh, mesh: pycc.ccMesh, unlinkPreviousOne: bool = True) None
- setTriangleIndex(self: pycc.ccSubMesh, localIndex: int, globalIndex: int) None